Accounts Identification by SSN/Tax ID
Matching Customers with Accounts
When building an application you have many data relationships to consider. An important one is between advisors, customers and their accounts. Advisors have customers and those customers have accounts.
Let’s briefly review the parts of this problem:
- You have advisor users (and presumably database records for them) in your system
- You have customer data (and presumably database records for them) in your system
- You have accounts data from the BridgeFT WealthTech API in your system
Assuming you can associate your advisor users to their respective customer records, the final challenge is:
“How can I find all the accounts from BridgeFT that belong to an advisor’s customer?”
One misconception is that the relationship of accounts to their customers exists in the custodian. This is not true. Custodians provide accounts and account ownership information. This is commonly known as “Name and Address” data. This is basic first name, last name, address, city, etc. These data points often don’t get delivered cleanly in their own fields, but rather get formatted into name and address lines along with account type information etc. This varies by custodian, making it even more complicated. It’s a bit of a parsing nightmare. Finally, name and address data can be outdated account by account, custodian by custodian. So attempting to match customers to their accounts using their address is both challenging and error prone.
There is, however, a data point that can be used with greater success: Social Security Number (SSN) or Tax Identification Number (Tax ID). Searching for all accounts that have the SSN/Tax ID of your customer will typically yield a more accurate list of accounts.
Here’s a typical workflow:
- An advisor locates a customer profile in your system (UI)
- The advisor searches for accounts that have the customer’s SSN (either the advisor inputs the SSN or your system has it in the database)
- The advisor is presented with a list of accounts along with name and address details for review
- The advisor selects all accounts the believe to truly belong to the customer
- The selected accounts are associated with the customer record in your system
Step 3 is an important step to consider. If you think there is a risk of linking the wrong account to a customer, visual verification by advisor is a good idea.
To assist you in overcoming this problem, BridgeFT provides a set of APIs to find and match all accounts using a unique SSN or Tax ID.
BridgeFT's Solution
The WealthTech API offers an accounts api that delivers a list of all your advisor's accounts. Among the data points delivered is a unique “tax_id_token” per account. For security purposes, we do not deliver the SSN in clear text. The “tax_id_token” represents a tokenized version of the SSN.
Additionally there is an api endpoint that allows you to retrieve a “tax_id_token” given clear text SSN string. Using the combination of these 2 api endpoints you can orchestrate the above workflow to match your advisor’s customers to accounts that have their SSN.
Before Starting Development
While most of the custodians provide Tax IDs in their data feeds, for security policy reasons some may not supply this information automatically. To ensure you receive the proper tokens associated with the identifiable information, please make sure that the release of unmasked SSN information is authorized at the time of executing custodian Release-of-Information (ROI).
For Schwab Authorization
Please submit a request to enable the tax identification data in your data feed by calling Advisor Platform Support (APS) team at 800-647-5465. If an advisor or a firm is sending BridgeFT multiple master accounts, each master account number needs to be requested during that time. Schwab requires that a separate request is completed each time a new master account is authorized.
For Other Custodians
Please reach out to your custodian for more details on how they handle and disclose Tax IDs and make sure this data is allowed to be sent to BridgeFT.
BridgeFT’s Supported Custodians
Below is the full list of BridgeFT’s integrated custodians, and Tax IDs data potential availability:
Custodian ID in BridgeFT | Custodian Name | Is Tax ID Supplied by default? |
---|---|---|
APX | Apex Fintech Solutions | Yes |
DST | DST Systems | Yes |
FPR | Fidelity 401K | No |
IBK | Interactive Brokers | No |
NFS | Fidelity Investments | Yes |
PER | Pershing | Yes |
SWB | Charles Schwab | Yes, but you have to submit the disclosure request |
EGB | Eaglebrook | Yes |
MLT | Millennium Trust | No |
TIA | TIAA Financial Services | Yes |
If you noticed that tax_id_token
field in the Accounts API responses still be empty, like below:
{
"id": 216962,
"display_name": "Mr. John Smith",
"name": "John Smith",
"custodian": "APX",
"acct_type": "SEP-IRA",
"number": "39DEMO76777",
"status": "funded",
.
.
.
"tax_id_token":
}
Please make sure you allowed your custodian to provide this information to BridgeFT by submitting the ROI request as per instructions above.
Implementation Approaches
These are generalized implementation approaches to provide some ideas on how you might connect advisor customers to their accounts via SSN. Your approach may vary based on your application and needs.
1. Advisor UI Search Approach
- Using the
/v2/account-management/accounts
endpoint, fetch and store all the accounts in your database - Present a UI that allows the advisor to search for accounts given a customer record
- Using the customer’s clear text SSN, fetch the
tax_id_token
from the/v2/account-management/tax-id/{taxid}
endpoint - Query your database for accounts that have a matching
tax_id_token
value - Present the list of accounts along with additional name and address details (i.e. first/last name, address, etc.) for advisor review
- The advisor visually inspects and selects accounts believed to belong to the customer
- Save the association of the selected accounts to the customer
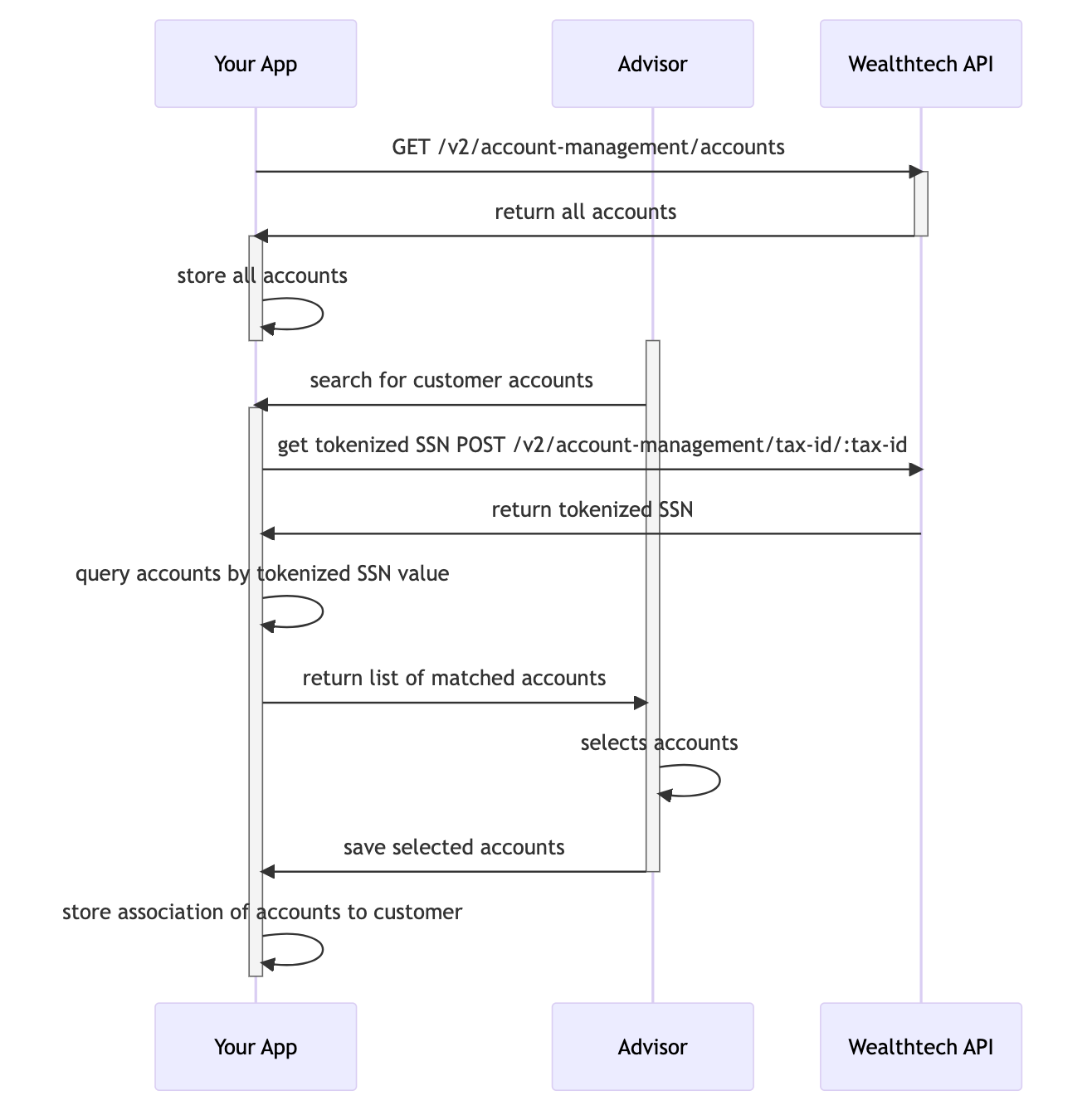
Requests Examples
Fetch List of Accounts from BridgeFT
Request:
curl --location --request GET 'https://api.bridgeft.com/v2/account-management/accounts?pager.limit=10000&pager.page=1' \
--header 'Authorization: Bearer {YOUR_AUTH_TOKEN}'
Response:
{
"id": 216961,
"display_name": "Mr. Niko McDermott DVM SEP-IRA",
"name": "Mr. Niko McDermott DVM",
"custodian": "SWB",
"acct_type": "SEP-IRA",
"number": "39DEMO76701",
"status": "funded",
.
.
.
"tax_id_token": "mmVoP94sN2bybwkm2JYRtY"
}
Fetch Tokenized Tax ID for the selected Account
To exchange a single Tax ID, for its tokenized representation, the Tax ID is provided in the path parameters of the API request.
Endpoint: /v2/account-management/tax-id/:taxid
Path Parameter: 000-00-0000
or 000000000
Request:
curl --location --request POST 'https://api.bridgeft.com/v2/account-management/tax-id/000-00-0002' \
--header 'Authorization: Bearer {YOUR_AUTH_TOKEN}'
The Tax ID string is a mandatory to input in this endpoint. It allows to contain dashes (-
), but are required to be only digits 0-9, with length no longer than 10 digits. Endpoints will return 400 Bad Request
error in case of missing or improperly formatted input.
Response:
"Pr7myKRzgennb2qGe32i3t"
2. Bulk Auto-Matching Approach
- Using the
/v2/account-management/accounts
endpoint, fetch and store all the accounts in your database - Present a UI that allows the advisor to search for accounts given a multiple customers records
- Using the customers clear text SSNs, fetch the Tokenized Tax IDs from the
/v2/account-management/tax-id
endpoint - Query your database for accounts that have a matching tokenized tax ids values
- Present the list of accounts along with additional name and address details (i.e. first/last name, address, etc.) for advisor review
- The advisor visually inspects and selects accounts believed to belong to the selected customers
- Save the association of the selected accounts and customers
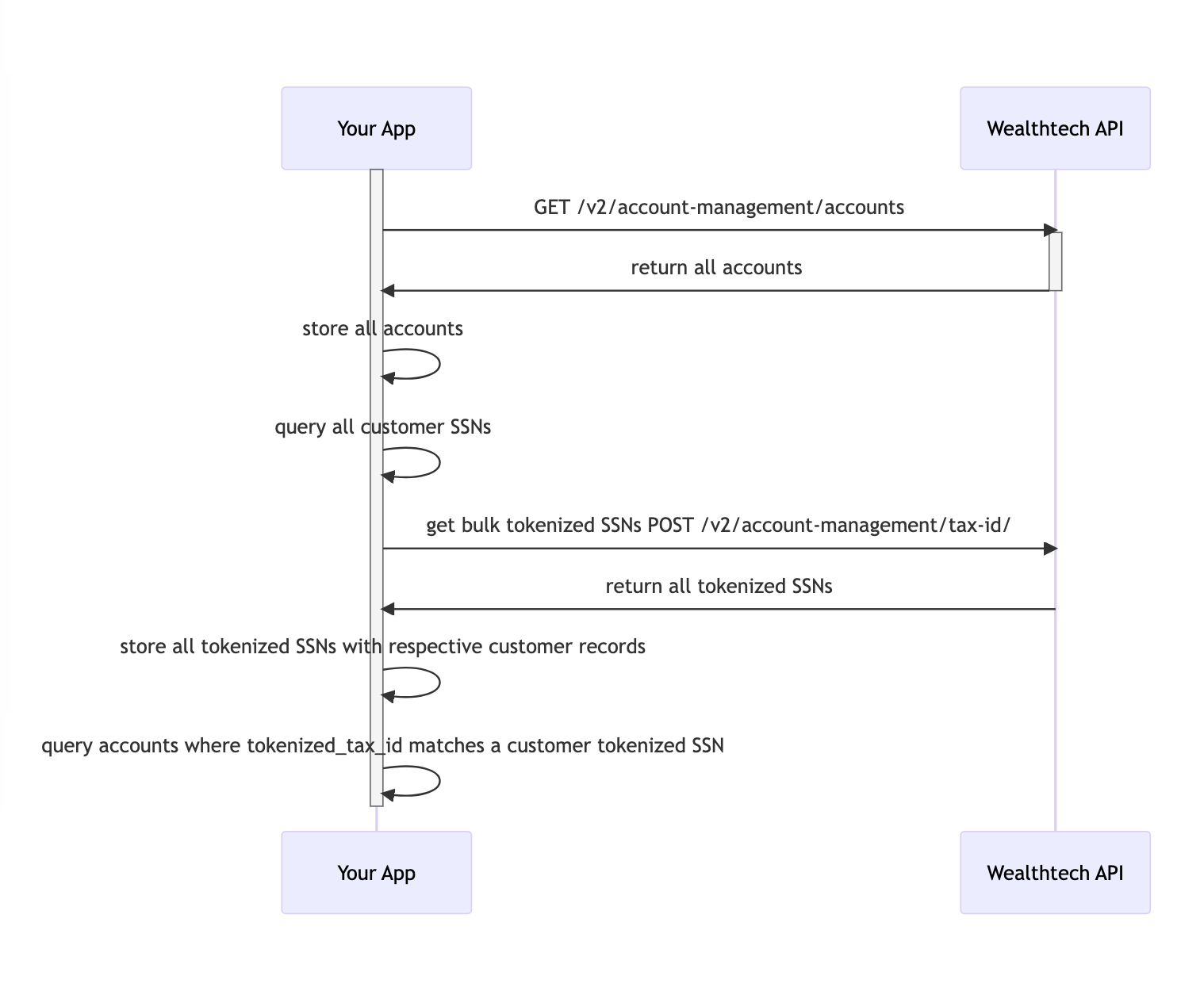
Requests Examples
Fetch bulk of Tokenized Tax IDs for the selected Accounts
To exchange multiple Tax IDs for their tokenized representations, the Tax IDs will be provided in json array in the body of the API Request.
Endpoint: /v2/account-management/tax-id
Request:
curl --location --request POST 'https://api.bridgeft.com/v2/account-management/tax-id' \
--header 'Authorization: Bearer {YOUR_AUTH_TOKEN}' \
--header 'Content-Type: application/json' \
--data-raw '[
"000-00-0002",
"000-00-0003",
"000-00-0004"
]'
Response:
[
"Pr7myKRzgennb2qGe32i3t",
"oSSiMxJFz6EjUotcu4qP4b",
"nMD9VtBVH9nD2d4RBwxLDd"
]
Match Real and Tokenized Tax IDs
The output order of the tokenized representations in the API response will always match the input order of the Tax IDs provided in the request. So when matching returned tokens to the real Tax IDs just follow the input ordering so that the result can be as following:
Input Tax ID | Matching Tokenized Representation |
---|---|
000-00-0002 | "Pr7myKRzgennb2qGe32i3t" |
000-00-0003 | "oSSiMxJFz6EjUotcu4qP4b" |
000-00-0004 | "nMD9VtBVH9nD2d4RBwxLDd" |
This step is supposed to be performed on your side because BridgeFT doesn’t store real Tax IDs and doesn’t expose it in API responses.
SSN/Tax IDs for Testing
Below are test accounts and fake SSN/Tax ID you can use for testing purposes:
custodian | account_number | ssn / tax id |
---|---|---|
SWB | 39DEMO06135 | 000000002 |
SWB | 39DEMO00806 | 000000003 |
SWB | 39DEMO09170 | 000000004 |
SWB | 39DE800096 | 000000006 |
SWB | 39DEMO06905 | 000000007 |
SWB | 39DEMO05997 | 000000008 |
SWB | 39DEMO06403 | 000000009 |
SWB | 39DEMO09423 | 000000010 |
Updated over 1 year ago