Authentication Guide
BridgeFT WealthTech API requests require authentication in order to access data. This guide will help you setup authentication in 2 simple steps.
What You Will Need to Get Started
You will need your client_id
and client_secret
to generate your access token.
BridgeFT will provide you with two pairs of credentials (client_id
and client_secret
), one set for our sandbox
environment and the other for our production
environment.
The Sandbox environment provides sanitized data for development and testing purposes. Accounts and portfolio data in sandbox is not "live". The Production environment instead consumes "live" data directly from BridgeFT's connected custodians.
The client_id
is an application's public identifier that is safe to be logged, used in communicating with support, and is used by BridgeFT to log and track API usage. The client_secret
is a confidential key that is strictly used in exchange for access tokens along with the client_id
. This secret should never be displayed or shared publicly.
Step 1. Get an APIs Access Token
Generate Basic Authorization string
BridgeFT requires the client credentials to be Base64 URL-encoded and sent via the Basic authentication pattern, per RFC 6749.
The unencoded string combines the client_id
and client_secret
with a single colon (:) separating the two like: <CLIENT_ID>:<CLIENT_SECRET>
. For example, if the client_id
is "BridgeFT" and the client_secret
is "WealthTech", the undecoded authorization header will appear as: BridgeFT:WealthTech
.
You need to encode this string in Base64 and provide it as an authorization header to get the access token:
Authorization: Basic QnJpZGdlRlQ6V2VhbHRoVGVjaA==
Feel free to use Auth Token API Reference page to generate Base64 authorization header, as on the following example:
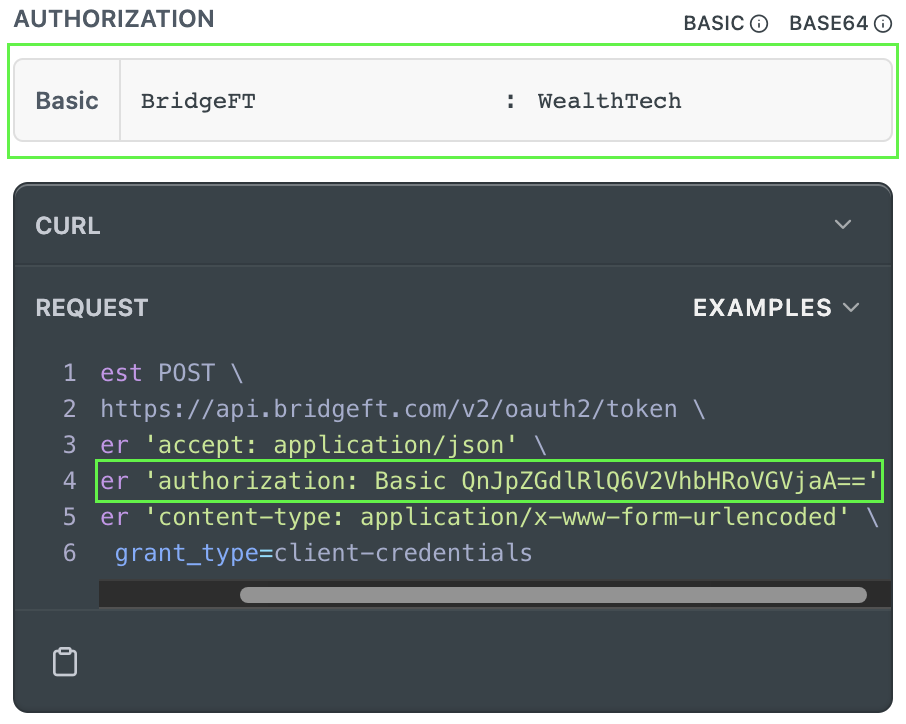
Get an Access Token
Now, having the authorization header, all that is required is to send a POST request to Get an Auth Token API.
Request Example
curl --request POST \
--url 'https://api.bridgeft.com/v2/oauth2/token' \
--header 'Authorization: Basic QnJpZGdlRlQ6V2VhbHRoVGVjaA==' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials'
Response Example
A successful response from the Access Token API:
{
"TokenType": "Bearer",
"ExpiresIn": 3600,
"AccessToken": "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsIng1dCI6Ik1uQ19WWmNBVGZNNXBP..."
}
Where:
Parameter | Description |
---|---|
AccessToken | Token which the application uses to access secured API resources. The access token is a JSON web token (JWT), encrypted using RS256 algorithm. |
ExpiresIn | The amount of time, in seconds, that the access token is valid for. |
TokenType | Indicates how the token should be used in the Authorization Header in any other APIs calls. This will always be Bearer . |
Step 2. Make Your First API Call
Now, having your valid access token, just provide it in the authorization header for any API requests you are making.
For example, if you wish to get an information about the particular customer's account send the following request:
curl --request GET \
--url 'https://api.bridgeft.com/v2/account-management/accounts/1234?pager.limit=10&pager.page=1' \
--header 'accept: application/json' \
--header 'authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsIng1dCI6Ik1uQ19WWmNBVGZNNXBP'
Access Token Expiration and Refresh
Access tokens will expire 1 hour after they are issued. Any API requests made with expired access tokens, will receive an error with 401 Unauthorized
.
It's important that you manage token expiration and refresh your token when it expires.
Access Token Content
The access token can be inspected for additional details using the tools and libraries made available by jwt.io.
Below is an example of a decoded access token:
{
"app": {
"application_id": 1,
"application_name": "BridgeFT Sample Client",
"client_id": "BridgeFT",
"firm_ids": null,
"organization_id": 1
},
"aud": [
"bridgeft/api"
],
"exp": 1669849184,
"iat": 1669845584,
"iss": "api.bridgeft.com/v2/oauth2/token",
"sub": "BridgeFT"
}
Where:
Parameter | Description |
---|---|
application_name | The name of the application. |
client_id | The client_id of the application. |
firm_ids | The list of firm_ids scoped to the token. When null , the application will receive a data for all of its accessible firms. |
exp | The time the access token expires. |
iat | The time the access token was issued. |
iss | The URL the access token was issued from. This will always be "api.bridgeft.com/v2/oauth2/token" . |
sub | The receiver of the access token. This will always be the client_id of the authenticated application. |
That's all there is to it, you are now set to enjoy using BridgeFT WealthTech API!
Updated 7 months ago